In modern day web/mobile applications, using a web service to enhance the performance has been a vital factor, since web services provide APIs to facilitate retrieving data/resources from somewhere on Internet. Various protocols and architectures have been defined to implement very reliable, efficient Web Services.Out of such Web Services in this post I will focus on RESTful Web Services. Before Jumping into how to implement a RESTful Web Service, first let’s dive into the Architectural pattern behind this technology (i.e : REST Architecture).
REST Architecture…
REST stands for REpresentational State Transfer. This idea was first introduced by Roy Fielding in 2000 for his Ph.D. dissertation at the University of California, titled Architectural Styles and the Design of Network-based Software Architectures.

Imagine a typical client-server implementation which is connected across the Internet. Client will send a request to the server asking for something (ideally to return a web page) and the server will respond with the relevant web page. But in a case where the client require specific data (not a web page, just DATA), server responding with some page would not do the work. As a solution to this, REST Architectural style came into existence where it provides the privilege of requesting a particular resource using the standard HTTP protocol.
Basic Structure of REST Architecture…
REST Architectural Style has some constrains which distinguishes it from other Web Service Architectures. Let’s look at some of the basic features which must be implemented in a Web Service that adheres to REST Architecture.
- Client-Server Architecture : RESTful Web Services could only be implemented in systems which implements client-server architecture.
- Stateless : This means that for a given request made by a client to the server, the client information is not stored by the server because client information is irrelevant for HTTP (in other words, client’s state is not recorded).
- Uniform Resource Identifier (URI) : For every resource which is accessible through the internet, a mechanism to identify each resource should have been implemented. URI serves that purpose.
- Resource caching : When a client request for a particular resource again and again, instead of requesting it from the server we can keep that resource somewhere temporarily. This is called Caching and it reduces the incoming traffic on the server.
Benefits of RESTful Web Services…
Nowadays there are so many organizations which make use of RESTful web services to ensure that valuable information are accessible to users who need them. In addition to this feature, RESTful web services offer some significant features as mentioned below.
- Implemented with the help of HTTP : Hyper Text Transfer Protocol (HTTP) is the protocol we use in communicating via Internet. And REST make the complete use of HTTP to facilitate the communication aspect of its architecture, unlike SOAP which is a protocol itself.
- Less bandwith usage : Compared to SOAP, REST uses much lesser bandwith for communication.
- Usage of JSON : JSON is a very simple data format compared to XML and REST communications heavily use it. This increases the overall performance of whatever the application built on top of REST architecture.
- High Scalability : You can easily increase the number of servers behind a load balancer.
- Language Independent : You can have Java, PHP, Python, Node.js servers implementing a REST API and perform without requiring additional resources.
- Supporting multiple data formats : Not only JSON, it supports complex data formats such as XML as well. In addition HTML and plain-text types are also widely used.
How REST Actaully Works…
REST Architecture is really easy to understand since it only uses HTTP to communicate between client and server. Here the client will send a request to the server, asking for a resource (could be considered as an object for understanding purposes). In order to facilitate the communication between client and server, there is an intermediate (RESTful API) which presents various methods for the client to access the resources in server.
Now what is a resource? It is very much similar to objects in OOP world. It represents a particular distinguishable item which is on the server side. Each resource is identified by URI (Uniform Resource Identifier).
Resources could be interpreted back to the client in various formats. It could be XML, JSON or in the form of a web page (HTML). The representation of a particular resource in different ways based on the client request is the basis of the REST Architecture.
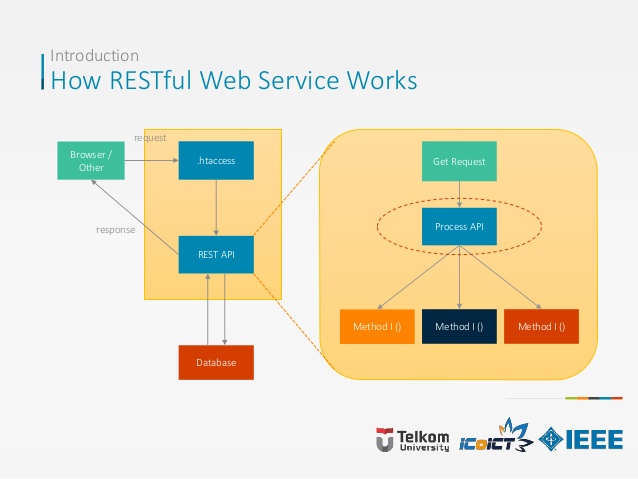
HTTP is the main driving force behind the success of RESTful Web Services. Hence we can make use of HTTP methods to facilitate the further facilitate the client-server communication. Following HTTP methods are adopted by RESTful Web Services to access resources from a particular REST API.
- GET : This is used to get a particular resource from the server side.
- POST : To enter some details to the given resource.
- DELETE : To remove the given resource.
- PUT : Responsible for modifying the content in that particular resource.
Let’s look at some of the examples for above mentioned HTTP methods.

API provides the methods to gain access to the resources belong to the server. In this case Github has provided several URI for each of the methods. Let’s see an example for how we can use HTTP GET method in accessing resources.

Here users represents a collection of users. Using the GET method, we can retrieve the resource users by typing it in the URL. Now what if we want the details of a particular user. Each user can be determined by the id. Let’s see how we can retrieve details of a particular user just by using the id.

Here the details of user with id = 1 is retrieved by just typing the id (1) after users/ . Note that same URL could be interpreted in different ways based on the HTTP method which is being used. Examples are listed below for the URL https://api.github.com/users/1
- POST : Insert user with id = 1
- PUT : Update user with id = 1
- DELETE : Delete the user with id = 1
Note : The operation type of PUT and DELETE are idempotent which means that irrespective of the number of times these methods are invoked, the result will always be the same. But POST method will result in a completely different output.
So What’s Next…
In this post the basics of RESTful Architecture and how it works has been covered upto a certain extent. Next we will look at the Group Project which will be developed for the Application Framework module in my degree program.