We already know that HTML and CSS are being utilized for building web pages and providing a nice design for the web pages respectively. When we talk about web development, it is safe to say that web pages also need to interact with users to provide dynamic yet rich content. To develop standalone applications or any other software there are so many programming languages such as Java, Python, C++ etc… Similarly there should be some sort of a programming language which ultimately enable web pages to be interactive and to provide dynamic content. To serve this purpose, there is something called JavaScript. Although JavaScript mainly acts as a web-based programming language, its scope is getting broader and broader everyday. We’ll dig more into that in the upcoming topics in this post.
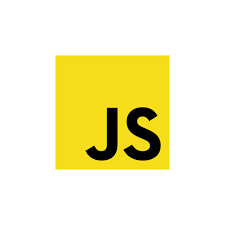
So What is JavaScript…?
To provide a more clear picture about JavaScript, it is a client-side scripting language which is implemented in Web browsers, in other words, you can do programming inside a HTML web page. When you use JavaScript inside a HTML page, you have to encapsulate them inside <script> tag.
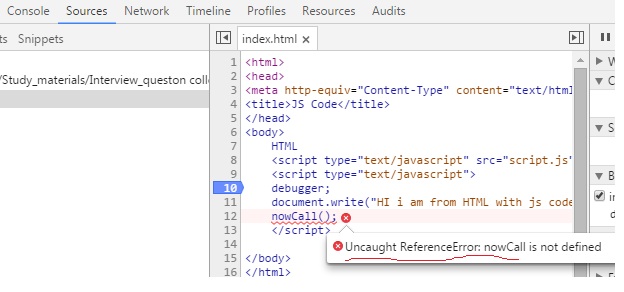
History of JavaScript…
The idea of JavaScript came into surface around 1995. Brendon Eich is considered as the author of JavaScript. Initially it was named as LiveScript, but was later changed into JavaScript. This name gave speculations about being a spin-off of the very popular Java language and turned out to be a marketing strategy adopted by Netscape (the first browser which implemented JavaScript) to popularize among the web community.

Why is JavaScript so Popular…?
JavaScript is supported by almost all the browsers out there. To be recognized as one of the most popular programming languages in the present day, JavaScript has some unique characteristics which makes it significant from its competitors.
- Fast : Implementation of JavaScript inside a browser using a JavaScript Engine makes running it really fast.
- Simplicity : JavaScript is very easy to learn and run. No need of installing additional libraries to run plain JavaScript.
- Platform-Independent : JavaScript runs without any problems in browser environment.
- Interoperability : JavaScript can be used inside languages such as PHP and Perl.
- Reduce server interactions : Through performing functions such as form validations, JavaScript can reduce the workload sent to a server, thus controlling the server traffic.
JavaScript and Java…
The prefix of “Java” in JavaScript will definitely force anyone into thinking that this is a sub-branch or a programming language developed using Java. But JavaScript is not even remotely related to Java…(This has been one of the frequently asked Questions in the Google Search Engine also).
Features in JavaScript…
JavaScript has features which are common to any programming languages such as variables, data types etc… But there are certain characteristics which are quite unique to JavaScript. We’ll see some of such common and unique features.
- Object-oriented : Yes, you can work with classes and objects in JavaScript as you would do in Java and any other language. Normally an object in JavaScript is defined within curly braces ({}) and you can create objects using new as well.
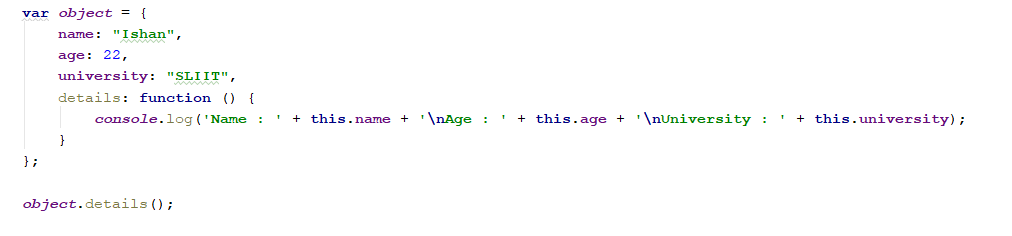
- Functional scoping and Block scoping : Initially only var keyword was allowed in JS which only enabled Functional scoping (Valid inside a given function). But after ECMAScript 2015, the introduction of let and const keywords made Block Scoping also possible (Valid inside a given scope).
- Prototypes : These are used by JS to simulate inheritance (will be discussed further).
- Weakly-typed : The data type of a variable is defined at the run-time, unlike in Java.
Applications of JavaScript…
Although JavaScript came into life only as a client-side scripting language initially, the range of applicability of JavaScript has been drastically broadened. Apart from being a scripting language, it now offers opportunity to implement server-side applications as well. In addition Mobile Applications also now can be created with the aid of advanced JavaScript tools. We’ll look into some of the main applications which uses JavaScript as its main language.
- NodeJS : This the best example for the use of JavaScript as a server-side applications. This was introduced in 2009 by Ryan Dahl.
- ReactJS : A JavaScript-based library which is predominantly used in creating interactive web pages.
- AngularJS: JavaScript-based open source front-end framework designed by Google.
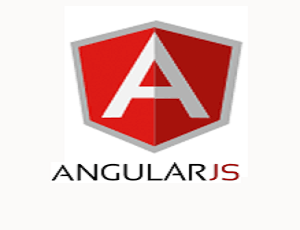
Basic JavaScript Concepts…
Earlier I talked about some unique features of JavaScript. This post will not be enough to cover every little thing about JS so I’ll be taking some of the points mentioned above and most important basic concepts that are available.
- Functions
- Objects and Creating Classes.
- ‘this’ concept
- Callbacks
- Closures
- Promises
Functions
Just like any other programming language, in JS also functions serve the purpose of executing a particular task once it is called in the program. There are two ways you can use functions in JavaScript.
- Declaring a function using ‘function’ keyword.
- Function as a Variable.
Examples for these two types are shown below.


In the second instance, you can call the function as printHello() since this variable is now considered as a function.
In addition to the above mentioned ways, function could be also be used in creating objects. That will be discussed under the next point.
Objects and Creating Classes
Previously I mentioned that JavaScript is an Object-oriented programming language thus making the concepts of classes and objects available. From the class definitions we can create multiple objects in JS as in any other OOP implemented language. But there is a slight difference where a function could be treated as a class and by calling that function we can create multiple instances of that class. Let’s see how that happens.
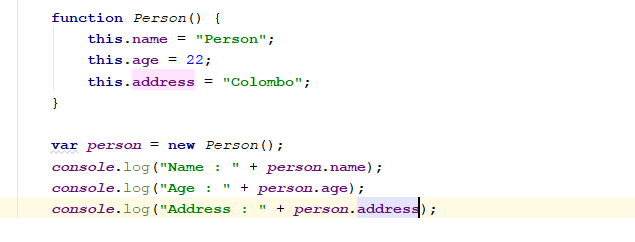

Note : To indicate that a particular function will be used as a Class, we give a name starting with an upper-case letter. To define properties inside this function, we use this keyword.
Inheritance in JavaScript could be achieved through prototypes. For that I’ll be using an example with two classes; Vehicle and Car where Car is inherited from Vehicle.
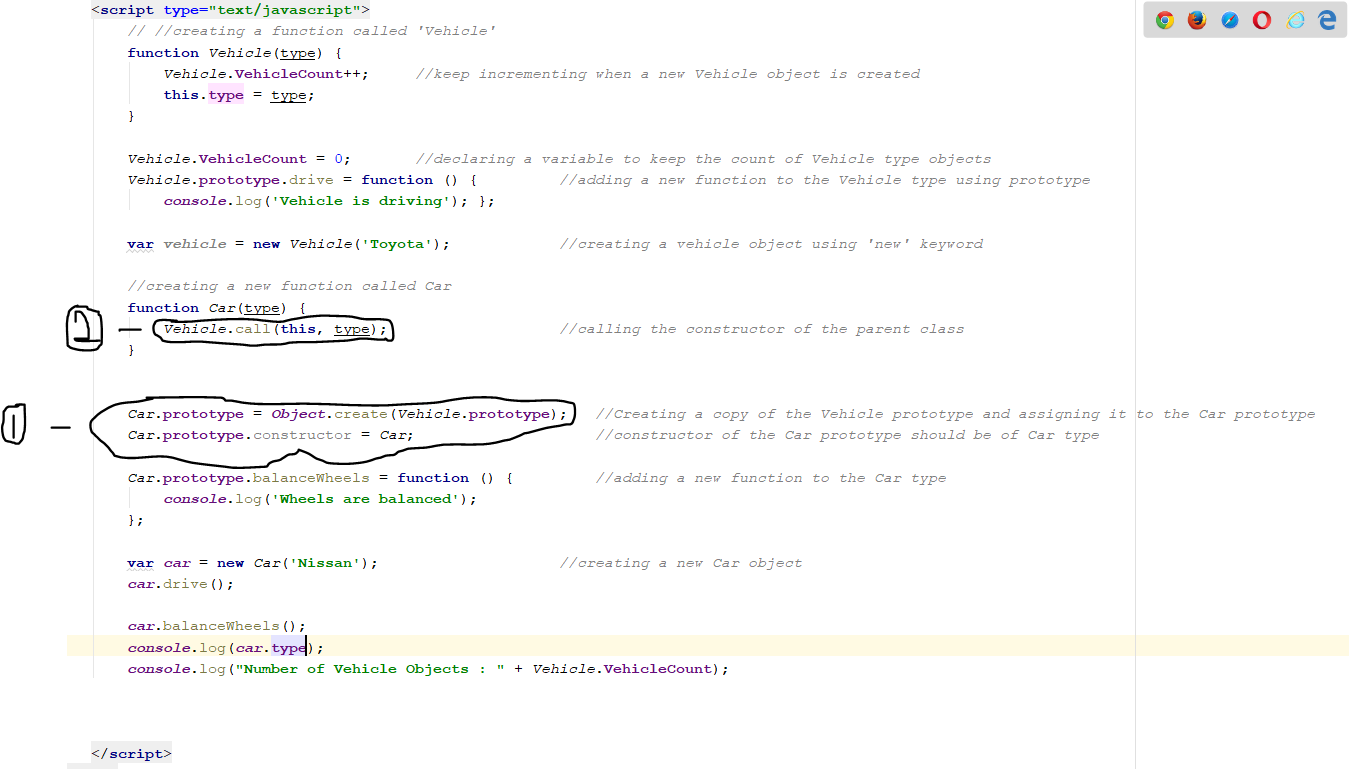

By default in JS, all the classes are inherited from a parent class called Object. Prototype of a class does not directly point to the Object class itself, but to a copy of it. This gives opportunity to add new behaviors to a class without affecting Object class (Because if we directly access the Object class do modifications, it will affect all the other classes inherited from it).
If we want to make Car as a child class of Vehicle, we need to follow two steps.
- Create a copy of the Vehicle prototype using Object.create() method and assign it to the prototype of the Car. Now whatever the modifications done in Car.prototype will not affect the Vehicle class. Then make sure that constructor of the prototype is of Car type.
- Inside the constructor of Car class, call the constructor of its parent class by using the call() method (parentclass.call()). You have to pass the reference of the context which it is being called (passing this as the first parameter).
‘this’ concept…
this keyword is used in many programming languages to refer the object context which it is being used. It carries the same idea in JavaScript also. But there are slight differences which could be seen here based on the context it is being referred from. Following example will show you exactly what that is.


When you print this object in the console you get the output as window, which means window is referred as this object in (1). The variable vehicleName belongs to the window object thus when you call the printVehicleNameOuter() function we still refer to that variable declared initially. But in the vehicle object there is another property declared with the same name. By assigning printVehicleNameOuter to printVehicleNameInner inside the object we could use it as a function of the vehicle object. In this case, now this refers to the variable inside the vehicle object, thus printing “Nissan” in line (3).
Let’s see what happens after the following modification.
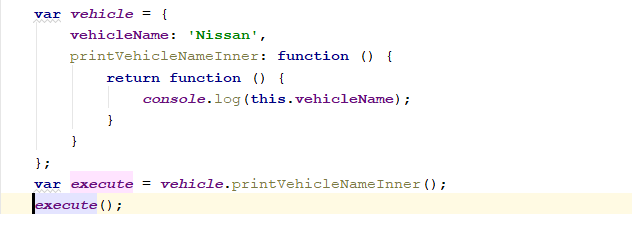

In this case, execute now holds a function returned by printVehicleNameInner(). So the way execute() now operates is similar to the function printVehicleNameOuter(), thus printing “Toyota”.
What if we want to print “Nissan” from the execute() method? We can achieve this using two methods.

Here we are passing a context inside the call() method. In other words, we are telling that consider whatever inside the vehicle as this. So now we will get “Nissan” instead of “Toyota”.

Or, we can link the context we want to the current context by using the bind() method. Here also we have to pass the context we desire as an argument.
Callbacks…
It is a well known fact that JavaScript runs as a single thread. So it does not create multiple threads to handle perform several tasks at the same time. JavaScript comes with a solution to simulate the multithreading aspect by introducing callback functions. Simply Callback is a function that we pass into another function as an argument, to execute the defined task after the execution of the main function.
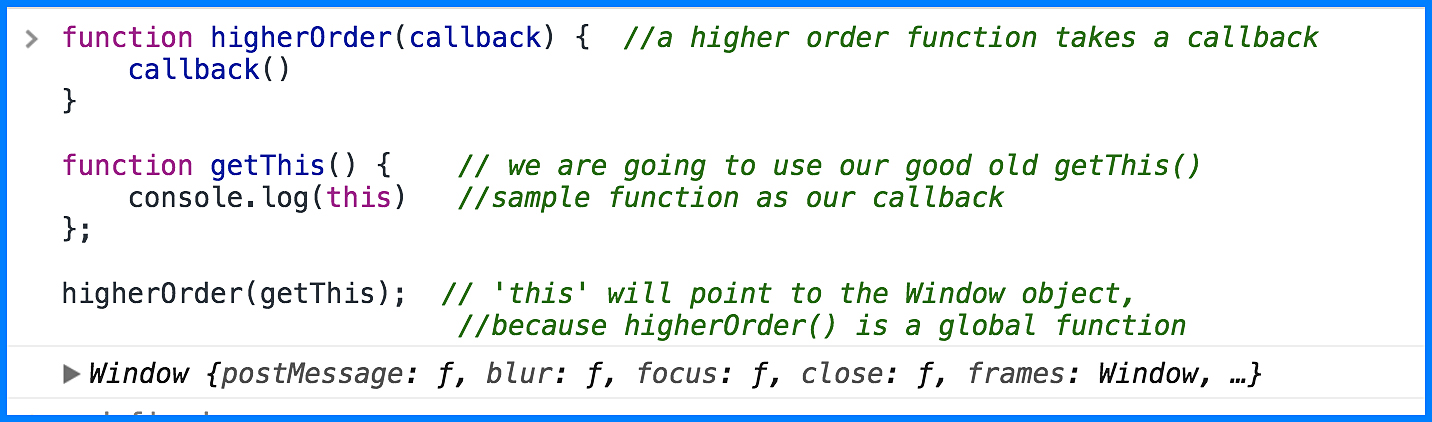
Let’s see a better example to find out how callback functions actually work.

cookAndDeliverFood() function will accept a callback function as a parameter. It will use a in-built function called setTimeout() function to do whatever the task specified in the callback function after 5 seconds. In the placeAnOrder() function, cookAndDeliverFood() is called by printing the customer number which has been already added as a parameter to the function.
Let’s see the output to understand how this code actually works.

As expected customer order numbers are printed sequentially. So we should get the respective output for each customer number sequentially as well. But the output is different to what we expected.

After 5 seconds all the order numbers are printed at the same time. How did this happen? That’s because callback functions are executed by the JavaScript Engine in a way that the client does not have to wait until it gets the response. It can carry out its own work. So in this case instead of customer number 1 process waiting until its response, other customer numbers can also be displayed.
Closures…
In simple words, Closures could be defined as returning a function from a function. The interesting thing about this concept is that the inner function encapsulates the variables of the outer function so that the variables defined could be accessed outside that function through this returned function. To get this idea clear, we’ll go through the following example.


In this case, tax1 contains the function returned by the taxPercentage(). But it also holds the value of the parameter passed to the taxPercentage() function. From the second step, tax1() now returns a value which is calculated using the tax percentage value included earlier.
Promises…
Promises have been introduced in order to cover up for some of the drawbacks of Callback functions. Using promises we can simplify the coding and enhance the readability as well. Let’s go through the code structure of promises.

A function that is passed with the arguments resolve
and reject
. The executor
function is executed immediately by the Promise implementation, passing resolve
and reject
functions (the executor is called before the Promise
constructor even returns the created object). The resolve
and reject
functions, when called, resolve or reject the promise, respectively. The executor normally initiates some asynchronous work, and then, once that completes, either calls the resolve
function to resolve the promise or else rejects it if an error occurred. If an error is thrown in the executor function, the promise is rejected. The return value of the executor is ignored.
Another use of the promises could be seen in fetch API as well.

First the response received from the url is converted into a JSON object inside the then function of the and returned as a promise object. In the next step, that returned promise object is taken inside another then function and printed into the console.
Through this article I was able to cover up some of the key features of JavaScript and few basic interesting concepts which are unique to JS.
Coming Up…
In the next post, the RESTful Architecture and how it can be used in web applications will be discussed in depth.